Database Optimization Techniques: Making your data fly
Databases are the backbone of modern applications, but without proper care, they can turn into sluggish bottlenecks. Whether you’re running a small app or a massive enterprise system, optimizing your database is key to keeping things fast, efficient, and scalable. Let’s explore some proven techniques to supercharge your database performance, from clever indexing to query tuning and beyond.
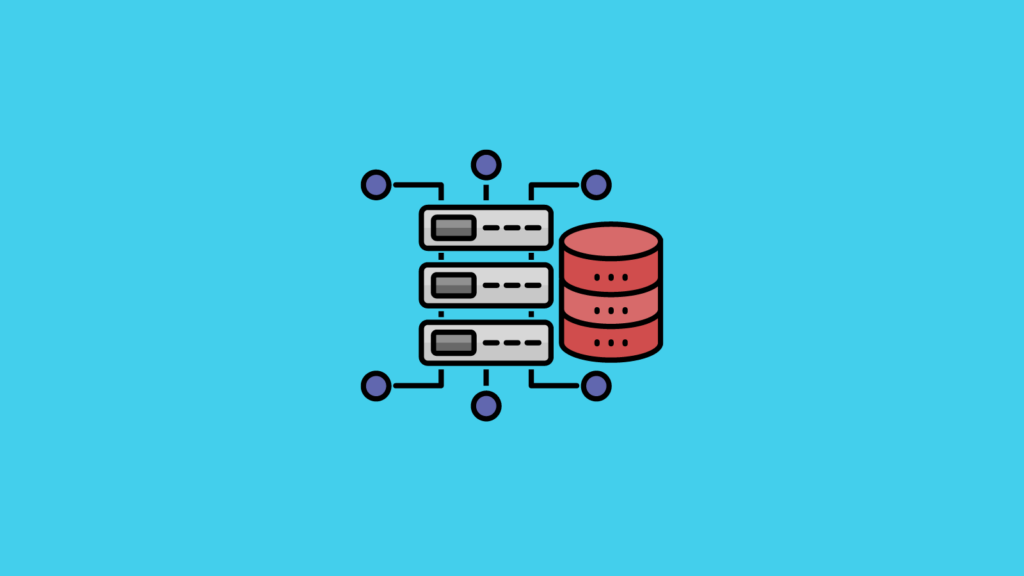
1. Mastering Indexing
Indexes are like shortcuts for your database, helping it find data without rummaging through every row. But they’re a double-edged sword—great for reads, tricky for writes. Here are two standout indexing strategies:
- Covering Indexes: These turbocharge queries by including all the columns a query needs right in the index itself. The database can grab everything it wants without touching the table data on disk—a move called an “index-only scan.” It’s a huge win for read speed, cutting down on slow disk operations. However, the trade-off is larger indexes, which slow down writes (inserts, updates, deletes) since every change updates the index too. Use them wisely for frequent, critical queries—just don’t overdo it.
-
Partial Indexes: Why index everything when
you only need a slice of the pie? Partial indexes let you
index a subset of rows based on a condition, like
WHERE status = 'active'
. This keeps the index lean and mean, boosting both read and write performance. Perfect for scenarios where you’re querying a specific chunk of data often, like recent orders or active users. -
Compound Indexes: Sometimes, a single-column
index isn’t enough. That’s where compound indexes come in.
These indexes combine multiple columns into one, letting you
optimize queries that filter or sort on several fields at
once. For instance, if you’re querying customers by
last_name
andbirth_date
, a compound index on both columns can handle it efficiently. Order matters here: the sequence of columns in a compound index determines which queries it can serve. A compound index on(last_name, birth_date)
works great forWHERE last_name = 'Smith' AND birth_date > '1990-01-01'
, but it’s less helpful if you’re only filtering onbirth_date
.
The trick with indexing? Balance. Monitor your workload, analyze query patterns, and avoid creating indexes you don’t need—too many can grind your database to a halt.
2. Query Optimization
Even the best indexes can’t save a poorly written query. Optimizing your SQL is like giving your database clear, concise directions instead of a treasure map. Here’s how:
-
Select Only What You Need: Avoid
SELECT *
—it’s lazy and pulls more data than necessary. Name the columns you actually want to reduce overhead. - Use Joins Wisely: Joins are powerful but pricey. Ensure tables are indexed on join columns, and avoid unnecessary multi-table mashups if a simpler query will do.
-
Filter Early: Push
WHERE
clauses and conditions as close to the source as possible to shrink the dataset before the heavy lifting starts.
Run an EXPLAIN
or ANALYZE
on your
queries (depending on your database system) to see how they’re
executed. You’ll spot inefficiencies—like full table scans—and
fix them fast.
3. Normalize (or Denormalize) Strategically
Database design impacts performance big time. Normalization—organizing data into separate tables to eliminate redundancy—keeps things clean and saves storage. But it can slow down reads due to extra joins. Denormalization, on the other hand, flattens data into fewer tables for faster reads at the cost of more storage and trickier updates.
- When to Normalize: Early in development or for write-heavy systems where data integrity is king.
- When to Denormalize: For read-heavy apps (like reporting dashboards) where speed trumps all.
Pick based on your app’s needs, and don’t be afraid to mix both in different areas.
4. Caching: Skip the Database Altogether
Why query the database every time when you can store hot data somewhere faster? Caching frequently accessed data—like user profiles or product details—in memory (using tools like Redis or Memcached) slashes response times.
- Query Caching: Store the results of complex, stable queries.
- Object Caching: Keep entire data objects in memory for quick retrieval.
Just remember to invalidate the cache when data changes, or you’ll serve stale info.
5. Partitioning and Sharding
As your database grows, splitting it up can keep performance steady.
- Partitioning: Divide a single table into smaller chunks (e.g., by date or region) within the same database. Queries hit only the relevant partition, not the whole table.
- Sharding: Spread data across multiple databases or servers. It’s more complex but scales horizontally for massive systems.
Both reduce the workload on any single piece of hardware, but they add complexity—use them when simpler tweaks aren’t enough.
6. Hardware and Configuration Tweaks
Sometimes the bottleneck isn’t the code—it’s the setup.
- Increase Memory: More RAM means more data and indexes can live in memory, dodging slow disk I/O.
- Tune Settings: Adjust database parameters (e.g., buffer pool size in MySQL, shared buffers in PostgreSQL) to match your workload.
- Upgrade Storage: Switch to SSDs if you’re still on spinning disks—faster I/O changes the game.
Wrapping Up
Database optimization isn’t a one-and-done deal—it’s an ongoing dance between reads, writes, and resource use. Start with indexing and query tuning, layer in caching or partitioning as needed, and keep an eye on how your app evolves. Test, measure (with tools like slow query logs or profiling), and tweak. A well-optimized database doesn’t just perform—it sings.