Go vs Python: A Developer’s Guide to Key Differences
When it comes to programming languages, Go (Golang) and Python are two heavyweights that often get compared. Python’s readability and versatility make it a favorite for beginners and data scientists, while Go’s simplicity and performance attract developers building scalable systems. Let’s dive into how these languages stack up across error handling, functions, classes and data structures, abstraction, concurrency, and performance—with a special spotlight on concurrency as a killer feature.
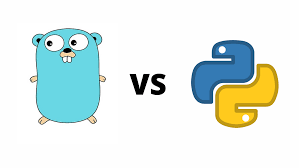
Error Handling: Explicit vs. Flexible
In Go, error handling is explicit and built into the language’s
DNA. Functions often return multiple values, typically a result
and an error. If something goes wrong, you check the error
manually with an if err != nil
block. It’s
straightforward but can feel repetitive. For example:
file, err := os.Open("example.txt")
if err != nil {
log.Fatal(err)
}
Python leans on exceptions with try
/except
blocks. This makes code cleaner when errors are rare, but it can
hide issues if you’re not careful:
try:
with open("example.txt") as file:
content = file.read()
except FileNotFoundError as e:
print(f"Error: {e}")
Go forces you to deal with errors upfront, which can prevent surprises in production. Python gives you flexibility but relies on you to anticipate exceptions. Go’s approach suits system-level reliability; Python’s fits rapid prototyping.
Functions: Simplicity vs. Dynamism
Go functions are minimalist. They take typed arguments, return typed values (sometimes multiple), and that’s it—no default parameters or keyword arguments:
func add(a int, b int) int {
return a + b
}
Python functions are more dynamic, offering default arguments,
keyword arguments, and variable-length argument lists
(*args
, **kwargs
):
def add(a, b=0):
return a + b
print(add(5)) # Works with one argument
print(add(5, 3)) # Works with two
Go’s rigidity keeps things predictable and fast; Python’s flexibility shines in scripting and experimentation.
Classes and Data Structures: Structs vs. Objects
Go uses struct
instead of traditional classes,
attaching methods via receivers. It’s simple but lacks
inheritance:
type Person struct {
Name string
Age int
}
func (p Person) Greet() string {
return "Hello, " + p.Name
}
Python embraces full OOP with classes, inheritance, and polymorphism:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
return f"Hello, {self.name}"
Go’s arrays, slices, and maps are lean and efficient; Python’s lists, dictionaries, and sets come with a richer ecosystem. Go prioritizes simplicity; Python prioritizes expressiveness.
Abstraction: Interfaces vs. Duck Typing
Go uses implicit interfaces for abstraction. If a type implements an interface’s methods, it satisfies it—no explicit declaration needed:
type Writer interface {
Write([]byte) (int, error)
}
Python relies on duck typing: if it has the right methods, it works:
def write_data(writer):
writer.write("data")
Go’s compile-time checks offer safety; Python’s loose approach offers speed.
Concurrency: Go’s Crown Jewel vs. Python’s Struggle
Concurrency is where Go pulls ahead as a game-changer, making it a selling point for modern software development. Go’s concurrency model, built around goroutines and channels, is lightweight, efficient, and intuitive—designed from the ground up to handle the demands of today’s multi-core, distributed systems.
A goroutine is a function that runs concurrently with others, managed by Go’s runtime rather than the OS. They’re incredibly cheap—thousands can run without breaking a sweat, costing just a few kilobytes of memory each. Compare that to OS threads, which can gobble up megabytes. Here’s a simple example:
func sayHello(ch chan string) {
ch <- "Hello from goroutine"
}
func main() {
ch := make(chan string)
go sayHello(ch) // Spin up a goroutine
fmt.Println(<-ch)
}
Channels provide a safe way to pass data between goroutines, avoiding messy locks or race conditions. Want to process a million tasks? Here’s how easy it scales:
func worker(id int, jobs <-chan int, results chan<- int) {
for job := range jobs {
results <- job * 2
}
}
func main() {
jobs := make(chan int, 100)
results := make(chan int, 100)
// Launch 10 workers
for w := 1; w <= 10; w++ {
go worker(w, jobs, results)
}
// Send jobs
for j := 1; j <= 100; j++ {
jobs <- j
}
close(jobs)
// Collect results
for r := 1; r <= 100; r++ {
fmt.Println(<-results)
}
}
This handles concurrency with elegance—no manual thread pools, no heavy synchronization. Go’s runtime schedules goroutines across CPU cores automatically, maximizing performance without developer overhead.
Python’s concurrency, by contrast, feels like a workaround. The
Global Interpreter Lock (GIL) in CPython prevents true parallelism
for CPU-bound tasks in threads. For I/O-bound work, threads or
asyncio
can help:
import asyncio
async def say_hello():
await asyncio.sleep(1)
print("Hello from asyncio")
asyncio.run(say_hello())
For CPU-bound parallelism, you’d turn to
multiprocessing
, which spawns separate
processes—effective but heavyweight compared to goroutines:
from multiprocessing import Process
def worker(num):
print(f"Worker {num} says hello")
processes = [Process(target=worker, args=(i,)) for i in range(10)]
for p in processes:
p.start()
for p in processes:
p.join()
Python’s model works, but it’s clunky. Processes don’t share memory easily, and the overhead is significant. Go’s goroutines and channels, meanwhile, make concurrency feel like a first-class citizen—simple to write, efficient to run, and scalable to millions of tasks. If you’re building a server handling thousands of connections or a system crunching data across cores, Go’s concurrency is a compelling reason to choose it over Python.
Performance: Compiled vs. Interpreted
Go’s compiled nature delivers C-like speed with garbage collection, perfect for high-throughput systems. Python, being interpreted, lags behind—though libraries like NumPy can boost specific tasks. Go’s edge is runtime efficiency; Python’s is development speed.
Final Thoughts
Go or Python? Go’s concurrency, performance, and reliability make it a powerhouse for scalable backends or distributed systems. Python’s flexibility, ease, and ecosystem shine for data science and rapid builds. Concurrency alone could tip the scales toward Go if your project demands efficient, parallel workloads. Why not master both? They’re perfect complements for different challenges.